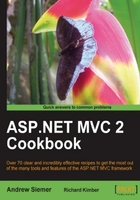
In this recipe, we will take a look at the new JsonResult
that comes with the MVC 2 framework.
We will use Json.NET (in the dependencies folder) to convert an object to JSON for us. And we will be using the Product
class from the Using magic strings and the ViewData dictionary recipe ofChapter 1. We will also be using NBuilder (also in the dependencies folder) to hydrate a Product
instance.
- Start a new ASP.NET MVC application.
- Add a reference to Json.NET.
- Add a reference to NBuilder.
- Create a new action named
GetJson
inside your HomeController. Instead of returning anActionResult
, we will specify that it will return aJsonResult
.HomeController.cs:
public JsonResult GetJson() { }
- Add the
Product
class to yourModels
folder (or create a newProduct
class). - Make sure that the
Product
class is set to[Serializable]
.Product.cs:
using System; namespace ExposingJSONFromJsonResult.Models { [Serializable] public class Product { public string Sku { get; set; } public string ProductName { get; set; } public string Description { get; set; } public double Cost { get; set; } } }
- Now we can specify our result by asking NBuilder to spit out an instance of
Product
. We can convert ourProduct
object into JSON using a built-in methodJson
.Note
When producing JSON in a
Get
method (as we are), you will also need to specify theJsonRequestBehavior
toAllowGet
. This is considered an XSS vulnerability though, so this is purely to render it in the browser as proof that it worked. Generally, you would want to use aPost
method with something like jQuery to get your JSON payload off the server.HomeController.cs:
public JsonResult GetJson() { return Json(Builder<Product>.CreateNew().Build(), JsonRequestBehavior.AllowGet); }
Short of using NBuilder, we have utilized the power of the new MVC 2 framework to generate a JSON result for us. It even took care of the serialization for us.
Be aware that exposing JSON via GET
requests on your site potentially opens you up to cross-site scripting attacks. However, not allowing GET
requests doesn't necessarily make you safe either—although it helps. Take a look at this discussion on Stack Overflow concerning this topic: http://stackoverflow.com/questions/1625671/what-is-the-problem-with-a-get-json-request.