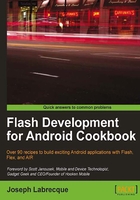
Accessing raw touchpoint data
Sometimes the predefined gestures that are baked into Flash Player and AIR are not enough for certain application interactions. This recipe will demonstrate how to access raw touch data reported by the operating system through Flash Player or AIR APIs.
How to do it...
To read raw touch data in your project, perform the following steps:
- First, import the following classes into your project:
import flash.display.StageScaleMode; import flash.display.StageAlign; import flash.display.Stage; import flash.display.Sprite; import flash.events.TouchEvent; import flash.text.TextField; import flash.text.TextFormat; import flash.ui.Multitouch; import flash.ui.MultitouchInputMode;
- Declare a
TextField
andTextFormat
object to allow visible output upon the device:private var traceField:TextField; private var traceFormat:TextFormat;
- We will now set up our
TextField
, apply aTextFormat
, and add it to theDisplayList
. Here, we create a method to perform all of these actions for us:protected function setupTextField():void { traceFormat = new TextFormat(); traceFormat.bold = true; traceFormat.font = "_sans"; traceFormat.size = 44; traceFormat.align = "left"; traceFormat.color = 0x333333; traceField = new TextField(); traceField.defaultTextFormat = traceFormat; traceField.selectable = false; traceField.mouseEnabled = false; traceField.width = stage.stageWidth; traceField.height = stage.stageHeight; addChild(traceField); }
- Set the specific input mode for the multitouch APIs to support touch input by setting
Multitouch.inputMode
to theMultitouchInputMode.TOUCH_POINT
constant. We will also register a set of listeners forTouchEvent
data in the following method:protected function setupTouchEvents():void { Multitouch.inputMode = MultitouchInputMode.TOUCH_POINT; stage.addEventListener(TouchEvent.TOUCH_MOVE, touchMove); stage.addEventListener(TouchEvent.TOUCH_END, touchEnd); }
- To clear out our
TextField
after each touch interaction ends, we will construct the following function:protected function touchEnd(e:TouchEvent):void { traceField.text = ""; }
- We can then read the various properties from the touch event to interpret in some way. Events such as pressure, coordinates, size, and more can be derived from the event object that is returned:
protected function touchMove(e:TouchEvent):void { traceField.text = ""; traceField.appendText("Primary: " + e.isPrimaryTouchPoint + "\n"); traceField.appendText("LocalX: " + e.localX + "\n"); traceField.appendText("LocalY: " + e.localY + "\n"); traceField.appendText("Pressure: " + e.pressure + "\n"); traceField.appendText("SizeX: " + e.sizeX + "\n"); traceField.appendText("SizeY: " + e.sizeY + "\n"); traceField.appendText("StageX: " + e.stageX + "\n"); traceField.appendText("StageY: " + e.stageY + "\n"); traceField.appendText("TPID: " + e.touchPointID + "\n"); }
- The result will appear similar to the following:
How it works...
Each touch point that is registered in the device has a number of specific properties associated with it. By registering a set of listeners to detect these interactions, we can read this data and the application can react appropriately. In our example, we are simply exposing these values via TextField
, but this would be the exact data we would need to build a pressure-sensitive gaming mechanic or some other custom gesture.
Note that on a device that allows more than one touchpoint, we will be able to read the data from both touchpoints using the same listener. Multiple touchpoints are differentiated by location on the stage and by touchPointID
. We would use these IDs to differentiate between touchpoints when devising complex gestures, or simply when we have the need to keep track of each touchpoint in a precise way.
There's more...
It is important to note that while Multitouch.inputMode
is set to MultitouchInputMode.TOUCH_POINT
that we will not be able to take advantage of the predefined gestures that Flash Player and AIR make available through the simplified gesture API. Setting the Multitouch.inputMode
to MultitouchInputMode.GESTURE
will allow us to take advantage of common, predefined gesture events within our application.