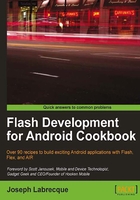
Using gestures to swipe a display object
Swipe is one of the most common gestures on Android devices, and with good reason. Whether flipping through a series of photographs, or simply moving between states in an application, the swipe gesture is something users have come to expect. A swipe gesture is accomplished by simply touching the screen and swiping up, down, left, or right across the screen quickly in the opposite direction.
How to do it...
This example draws a square within a Shape
object using the Graphics
API, adds it to the Stage
, and then sets up a listener for swipe gesture events in order to move the Shape
instance against the bounds of our screen in accordance with the direction of swipe:
- First, import the following classes into your project:
import flash.display.StageScaleMode; import flash.display.StageAlign; import flash.display.Stage; import flash.display.Sprite; import flash.display.Shape; import flash.events.TransformGestureEvent; import flash.ui.Multitouch; import flash.ui.MultitouchInputMode;
- Declare a
Shape
object which we will perform the gestures upon:private var box:Shape;
- Next, construct a method to handle the creation of our
Shape
and add it to theDisplayList:
protected function setupBox():void { box = new Shape(); box.graphics.beginFill(0xFFFFFF, 1); box.x = stage.stageWidth/2; box.y = stage.stageHeight/2; box.graphics.drawRect(-150,-150,300,300); box.graphics.endFill(); addChild(box); }
- Set the specific input mode for the multitouch APIs to support touch input by setting
Multitouch.inputMode
to theMultitouchInputMode.TOUCH_POINT
constant and register an event listener forTransformGestureEvent.GESTURE_SWIPE
events:protected function setupTouchEvents():void { Multitouch.inputMode = MultitouchInputMode.GESTURE; stage.addEventListener(TransformGestureEvent. GESTURE_SWIPE, onSwipe); }
- We can now respond to the data being returned by our swipe event. In this case, we are simply shifting the x and y position of our
Shape
based upon the swipe offset data:protected function onSwipe(e:TransformGestureEvent):void { switch(e.offsetX){ case 1:{ box.x = stage.stageWidth - (box.width/2); break; } case -1:{ box.x = box.width/2; break; } } switch(e.offsetY){ case 1:{ box.y = stage.stageHeight - (box.height/2); break; } case -1:{ box.y = box.height/2; break; } } }
- The resulting gesture will affect our visual object in the following way:
Note
Illustrations provided by Gestureworks (www.gestureworks.com).
How it works...
As we are setting our Multitouch.inputMode
to gestures through MultitouchInputMode.GESTURE
, we are able to listen for and react to a host of predefined gestures. In this example we are listening for the TransformGestureEvent.GESTURE_SWIPE
event in order to shift the x and y position of our Shape
object. By adjusting the coordinates of our Shape
through the reported offset data, we can adjust the position of our object in a way that the user expects.
We can see through this example that the offsetX
and offsetY
values returned by our event listener will each either be 1 or -1. This makes it very simple for us to determine which direction the swipe has registered:
- Swipe up: offsetY = -1
- Swipe down: offsetY = 1
- Swipe left: offsetX = -1
- Swipe right: offsetX = 1
There's more...
When reacting to swipe events, it may be a good idea to provide a bit of transition animation, either by using built in tweening mechanisms, or an external tweening engine. There are many great tweening engines for ActionScript freely available as open source software. The use of these engines along with certain gestures can provide a more pleasant experience for the user of your applications.
We might consider the following popular tweening engines for use in our application:
TweenLite: http://www.greensock.com/tweenlite/