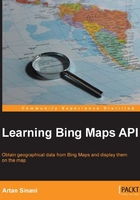
Chapter 2. Diving into Bing Maps AJAX Control Version 7
With the AJAX Control, we can add pins, lines, and polygons to the map. We can even add complex shapes, such as donut structures, if we load the Microsoft.Maps.AdvancedShapes
module. However, the library does not have built-in tools that allow the user to draw shapes manually, so let's create one.
We will build the code we wrote in the previous chapter. This means that we have an app.js
file, where we instantiate the map, and load the LearningTheme
module, which customizes the look and the behavior of the map.
We will create our tool as a module, and name it ShapeDrawing
. Just as we did in Chapter 1, Introduction to BING Maps AJAX Control Version 7, let's create a folder named shapeDrawing
, and a JavaScript file inside it with the same name. The module skeleton is simple, as shown in the following code:
(function() { var lbm = window.lbm || {}; lbm.ShapeDrawing = function(map) { this._map = map; }; Microsoft.Maps.moduleLoaded('lbm.ShapeDrawing'); })(this);
We declare the module under the namespace lbm
, so that it does not conflict with other library objects with the same name. The ShapeDrawing
function accepts only the map
argument, which is an instance of the Microsoft.Maps.Map
class, stored in the _map
instance variable.
At the end we make a call to Microsoft.Maps.moduleLoaded
, which turns the function into a loadable module by the AJAX Control.
To load the module on the page, we need to register it in the app.js
file we created in the previous chapter, which now looks as follows:
window.onload = function() { var container = document.getElementById('lbm-map'), resize = function () { container.style.height = window.innerHeight + 'px'; }; resize(); var map = new Microsoft.Maps.Map(container, { credentials: '[YOUR BING MAPS KEY]', center: new Microsoft.Maps.Location(39.554883, -99.052734), mapTypeId: Microsoft.Maps.MapTypeId.road, zoom: 4, showBreadcrumb: false, showDashboard: false }); Microsoft.Maps.registerModule('lbm.LearningTheme', 'learningTheme/learningTheme.js'); Microsoft.Maps.loadModule('lbm.LearningTheme', { callback: function() { var learningTheme = new lbm.LearningTheme(map); } }); Microsoft.Maps.registerModule('lbm.ShapeDrawing', 'shapeDrawing/shapeDrawing.js'); Microsoft.Maps.loadModule('lbm.ShapeDrawing', { callback: function() { var shapeDrawing = new lbm.ShapeDrawing(map); } }); window.onresize = function() { resize() }; };
When the AJAX Control loads the module, we create an instance of ShapeDrawing
, passing it a reference to the map.